SIP Client: How to Build a Basic SIP Client Using the SipJs Library
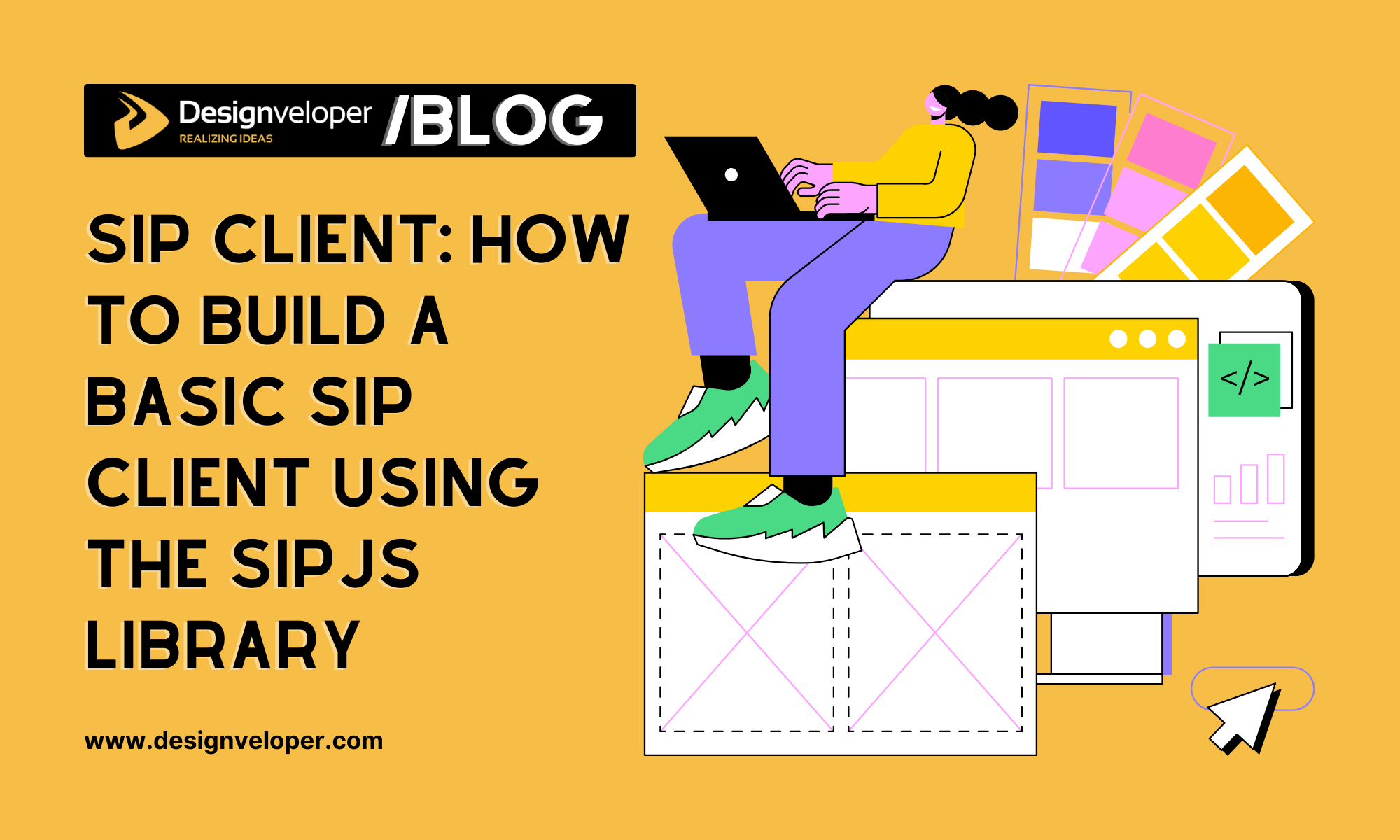
It is easy to build a SIP Client using the SipJs Library. The SIP Client is critical in the provision of real time communication over the internet. And the SipJs Library helps in the development of SIP clients by offering a sound framework for SIP signaling and WebRTC support. SipJs can help to create audio and video sessions with very little code and that is why it is so popular among developers.
Also, the SipJs Library provides several features such as user registration, session management, and real-time messaging. These features are essential for developing an effective SIP Client and the library supports all the popular browsers.
For instance, you can create a simple SIP Client that starts a video call with only a few lines of code with the help of SipJs. This simplicity cuts short the time and energy required in the development of the software. For further information, read the following article from your very own Designveloper.
Prerequisites
To build a basic SIP Client using the SipJs library, certain prerequisites are essential.
List of required tools and libraries (Node.js, npm, SipJs)
To develop a simple SIP Client using SipJs library, there are several tools and libraries required as follows: These tools help to make the development process as fluid and painless as possible.
Node.js
Node.js is a JavaScript runtime based on the Chrome’s V8 JavaScript engine. It enables developers to execute JavaScript on the server side and it is famous for its non-blocking, event-driven model that is suitable for building scalable network applications.
npm (Node Package Manager)
npm is the default package manager for Node. js, to handle dependencies and packages that are necessary for the project. With over 1.3 million packages on offer, npm makes it easy to install, update and manage libraries. For instance, to install the SipJs library, one can use the command npm install sip.js.
SipJs
SipJs is a Javascript library that helps developers create SIP clients for web applications. It supports SIP over WebSocket that makes it possible to use real SIP in web apps. SipJs is very light and simple to use but it has a very strong user API. It integrates well with other servers such as OverSIP, Kamailio, and Asterisk. A basic example of SipJs is the creation of the user agent and the initiation of the SIP call. The following code snippet demonstrates this:
var socket = new JsSIP.WebSocketInterface(‘wss://sip.myhost.com’);
var configuration = {
sockets: [socket],
uri: ‘sip:[email protected]’,
password: ‘superpassword’
};
var ua = new JsSIP.UA(configuration);
ua.start();
With these tools and libraries, one can easily develop a good SIP Client as explained in this paper. The combination of Node.js, npm, and SipJs offer a good starting point for building large-scale and dependable SIP applications.
Basic knowledge of JavaScript and web development
JavaScript is widely popular in web development. As per the recent survey, more than 97% of the websites are using JavaScript. This statistic shows how significant JavaScript is to current web development.
HTML and CSS knowledge is also required to work on the project. HTML organizes the content of the web while CSS decorates it. Along with JavaScript, they are the basic tools of web development.
For example, while developing a SIP Client, JavaScript can be used to manage user interactions and real-time communication. SipJs library helps in this by offering a reliable API for the SIP signaling. This library enables the developers to create audio and video sessions with less amount of code as shown below.
Furthermore, one must know the Document Object Model or DOM. DOM is the structure of a web page and JavaScript can change DOM to make the content dynamic. For instance, during a SIP call, JavaScript can change the user interface in real time.
Moreover, prior knowledge of WebRTC (Web Real-Time Communication) is helpful. WebRTC is used to provide peer-to-peer communication in web browsers which is required for SIP clients. The SipJs library uses WebRTC to enable the making of calls in audio and video.
Setting Up the Environment
Configuration of the environment for a SIP Client is very important. This step is important to make sure that the development process does not encounter any major hitches.
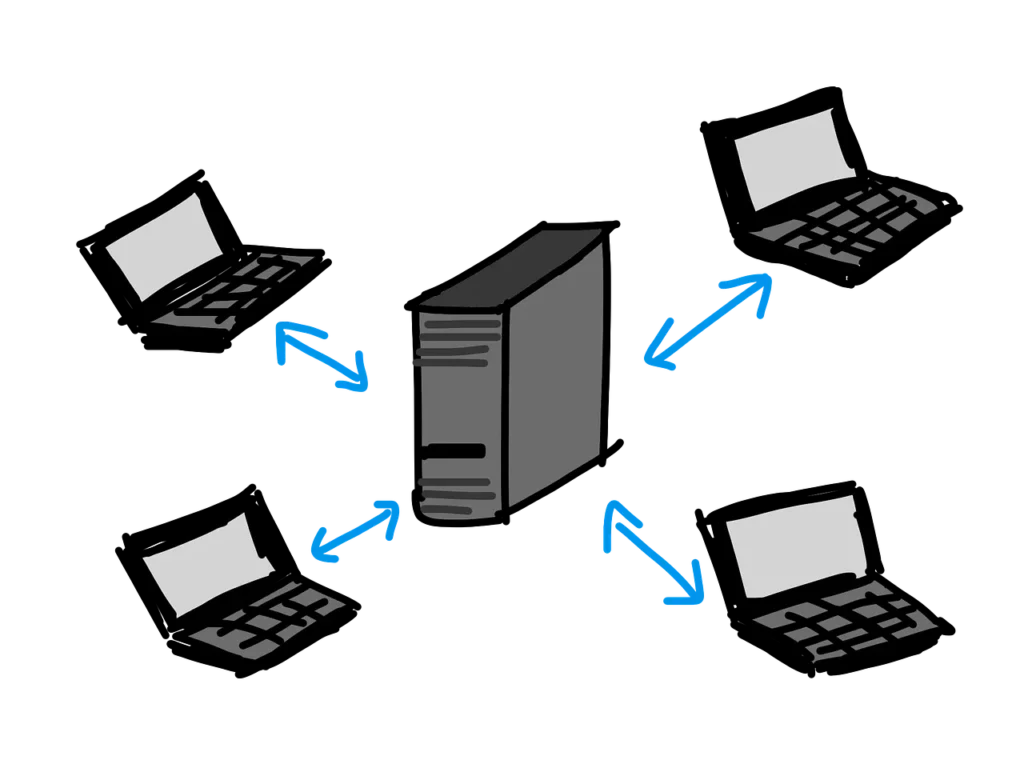
Installing Node.js and npm
To develop a SIP Client with the help of SipJs library, it is important to note that Node.js and npm are crucial. Node.js is a JavaScript runtime based on Chrome’s V8 JavaScript engine. npm is the package manager for Node.js, which is used to manage dependencies.
Step-by-Step Installation Guide
- Download Node.js: Go to the official Node.js website and download the installer for your operating system. Select the LTS version to get the most stability.
- Run the Installer: Open the downloaded file and follow the prompts. Accept the license agreement and choose the default settings. This will install both Node.js and npm on your computer.
- Verify Installation: Go to your command prompt or terminal. To check the installed versions, use the commands node -v and npm -v. This ensures that Node.js and npm are installed properly.
Using Node Version Manager (nvm)
To have better control over Node.js versions, use a Node version manager such as nvm. It enables the user to be able to work with different versions of Node.js and npm. This is beneficial in the sense that one can test an application on different versions.
- Install nvm: Just follow the instructions on the nvm GitHub page.
- Install Node.js with nvm: To install the latest version of Node, type the command nvm install node. js. You can also use version number to install a certain version of the software.
- Switch Versions: To select between installed versions of Node, use nvm use <version>.
Creating a new project directory
To begin developing a SIP Client with the help of SipJs library, the first thing to do is to create a new project folder. This directory will contain all the files and folders essential for the project.
First of all, you need to launch your terminal or command prompt. Go to the directory where you wish to create your project folder. In this case, you will use the mkdir command and follow it by the name of your project. For example, type mkdir sip-client-project and press ‘Enter’. This command forms a new directory with the name of sip-client-project.
After that, use the cd command to move into the newly created directory. Open the terminal and type cd sip-client-project and press enter. Now you are in your project directory.
In your project folder, create subfolders for various parts of your SIP Client. For example, you can make directories src for source codes, tests for test files, and config for configuration files. Now use the mkdir command again to create the following subdirectories. For instance, the user will type mkdir src tests config and hit the Enter key.
A good directory structure is a must. It makes a guarantee that all files are in their right places. Many developers use this practice and it is regarded as a standard practice in software development.
Also, you can develop a README.md file in the root of your project directory. This file will include details about your project including its use, how to create it and how to go about using it. To create this file, use the command touch README.md and press Enter.
Installing the SipJs library using npm
The following are the steps to install the SipJs library using npm. First, ensure that Node.js is installed on the system. Node.js is required to execute npm commands.
Secondly, open a terminal or command prompt on your computer or device. Switch to the directory of the project. This is where the SIP Client will be developed.
To initialize a new Node.js project, one needs to type the following command:
npm init -y
This command creates a package.json file. The file is a metadata of the project.
Now, install the SipJs library by running:
npm install sip.js
This command installs the SipJs library which is used in the application. It also appends the library to the ‘dependencies’ part of the ‘package.json` file.
After installation, ensure that the library is well installed. Check the `node_modules` directory. The directory should contain a folder named ‘sip.js`.
Last but not the least, import the SipJs library into the project files. Use the following code snippet:
const SIP = require(‘sip.js’);
This code introduces the SipJs library to be used in the project.
Building the Basic SIP Client
It is easy to build a simple SIP client using the SipJs library as shown below. The market for SIP clients is expanding at a fast pace and the global SIP trunking market is expected to touch US$30.22 billion by 2027. This growth is due to the need for more efficient, reliable and cost effective telecommunications solutions.
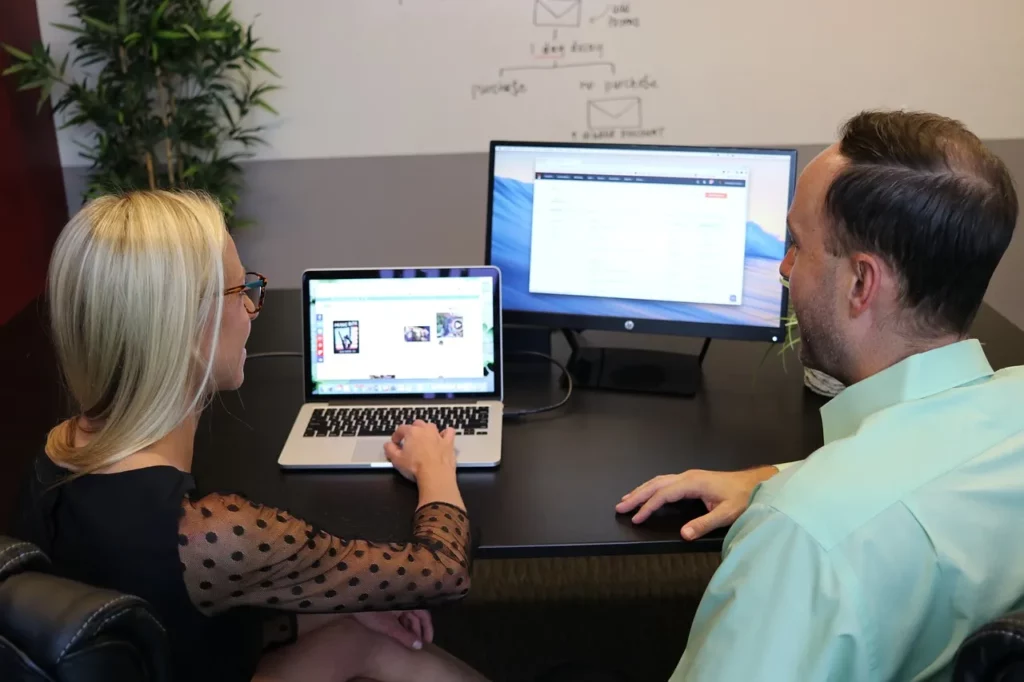
Initializing the SipJs library
When developing a basic SIP client, it is mandatory to initialize the SipJs library to begin with. This step creates the context for the SIP client.
First of all, you need to download and install the SipJs library. Use the following command:
npm install sip.js
After installation, the following modules are required to be imported:
import { UserAgent } from ‘sip.js’;
Next, configure the UserAgent. This entails configuration of the SIP server and the user credentials. Here is a sample configuration:
const userAgent = new UserAgent({
uri: UserAgent.makeURI(‘sip:[email protected]’),
transportOptions: {
server: ‘wss://sip.example.com’
}
});
The uri is the SIP address of the user. The server sets the WebSocket server for the SIP signaling.
Once configured, start the UserAgent:
userAgent. start(). then(() => {
console. log(‘UserAgent started’);
});
This code is used to start the SIP client and also links it to the server.
Setting up the configuration options (SIP server, user credentials)
In order to create a simple SIP client with the help of the SipJs library, the configuration options are of great importance. The first step is to describe the SIP server. SIP server is the main entity that controls all the SIP messages that are exchanged between the two parties.
Second, set the user credentials. User credentials refer to the username and password that is needed to login with the SIP server. For instance, if using a service such as Twilio, the credentials will be found in the Twilio console. Make sure these credentials are well protected and not embedded in the application.
Here is a sample configuration:
const configuration = {
uri: ‘sip:[email protected]’,
transportOptions: {
wsServers: [‘wss://sipserver. com/ws’],
},
authorizationUser: ‘username’,
password: ‘password’,
};
This configuration defines the URI, the transport options and the authentication details. The wsServers parameter defines the WebSocket server for the SIP signaling. WebSocket guarantees real-time communication, which is required for a SIP client.
Also, there is the need to handle the registration with the SIP server. Registration enables the SIP client to be able to receive calls from other parties. The following code snippet demonstrates how to register:
const userAgent = new SIP.UA(configuration);
userAgent.start();
This code generates a new user agent and initiates the registration procedure. The user agent is responsible for all signaling using SIP and media sessions.
Creating a simple user interface (HTML/CSS)
For constructing a basic SIP client, it is necessary to have a graphical user interface.
First, let’s create an HTML file. This file will define the layout of the SIP client. Semantic HTML elements should be used for improved accessibility. For instance, you should use <header>, <main>, and <footer> elements.
<!DOCTYPE html>
<html lang=”en”>
<head>
<meta charset=”UTF-8″>
<meta name=”viewport” content=”width=device-width, initial-scale=1.0″>
<title>SIP Client</title>
<link rel=”stylesheet” href=”styles.css”>
</head>
<body>
<header>
<h1>SIP Client</h1>
</header>
<main>
<div id=”call-controls”>
<button id=”call”>Call</button>
<button id=”hangup”>Hang Up</button>
</div>
</main>
<footer>
<p>© 2024 SIP Client</p>
</footer>
</body>
</html>
After that, apply styles to the interface using CSS. It enhances the readability of the text when the design is clean. Use CSS to position elements and to set margins. For instance, use Flexbox for layout.
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
display: flex;
flex-direction: column;
min-height: 100vh;
}
header, footer {
background-color: #333;
color: white;
text-align: center;
padding: 1em 0;
}
main {
flex: 1;
display: flex;
justify-content: center;
align-items: center;
}
#call-controls {
display: flex;
gap: 1em;
}
button {
padding: 0.5em 1em;
font-size: 1em;
cursor: pointer;
}
This example shows a simple SIP client interface. The HTML provides structure. The CSS improves the aesthetic value of the site. Combined, they form a friendly SIP client for use by the users.
Implementing the basic functionalities (registering, making a call, receiving a call)
There are several steps that need to be followed when creating a simple SIP client with the help of the SipJs library. First, the client has to sign up with a SIP server.
To register a SIP client, the user agent instance has to be created. This instance is responsible for the communication with the SIP server. Use the following code snippet to register:
const userAgent = new SIP.UserAgent({
uri: ‘sip:[email protected]’,
transportOptions: {
wsServers: [‘wss://sipserver.com’]
}
});
userAgent.start();
This code sets the user agent and makes it join the SIP server. The `start()` method is used to register the client.
Once the client has registered, he or she is able to make calls. Use the `invite` method to initiate a call:
const target = ‘sip:[email protected]’;
const inviter = new SIP.Inviter(userAgent, target);
inviter.invite();
Here is the example of how to call a target SIP address: The `invite` method initiates the call request.
For the client to be able to receive calls, the client has to wait for call requests. Use the following code to handle incoming calls:Use the following code to handle incoming calls:
userAgent.delegate = {
onInvite(invitation) {
invitation.accept();
}
};
This code creates an event handler for calls coming in. The `onInvite` method takes the call without further ado.
These basic functionalities are implemented so that the SIP client is able to register, make and receive calls effectively.
Conclusion
In conclusion, it can be stated that the knowledge of the SipJs library for creating SIP clients can be beneficial for a developer. It creates possibilities in the expanding VoIP segment. In this article, the steps to follow in order to design good SIP clients are highlighted and these should be followed by developers in order to come up with good SIP clients.
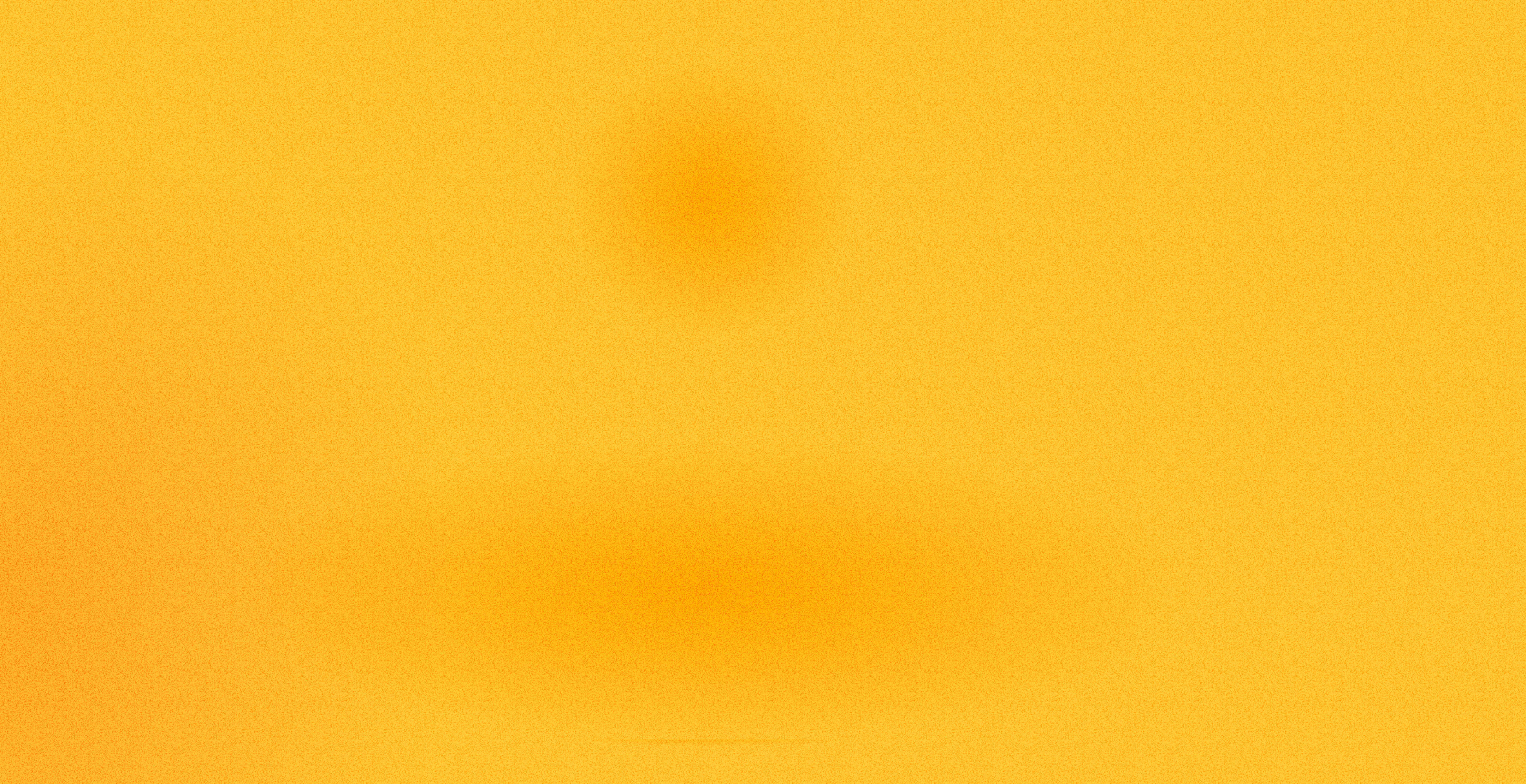
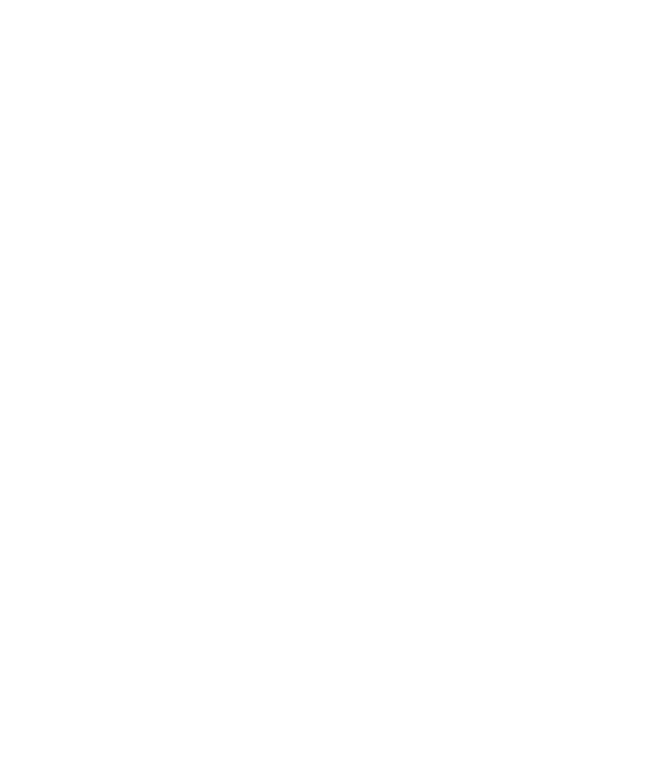
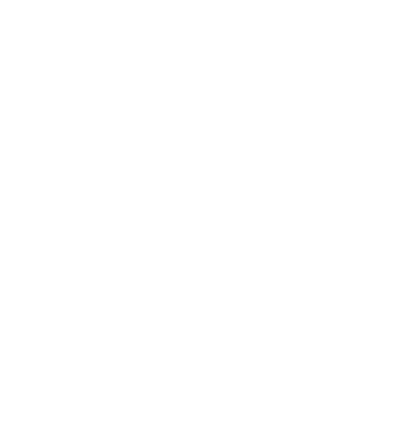
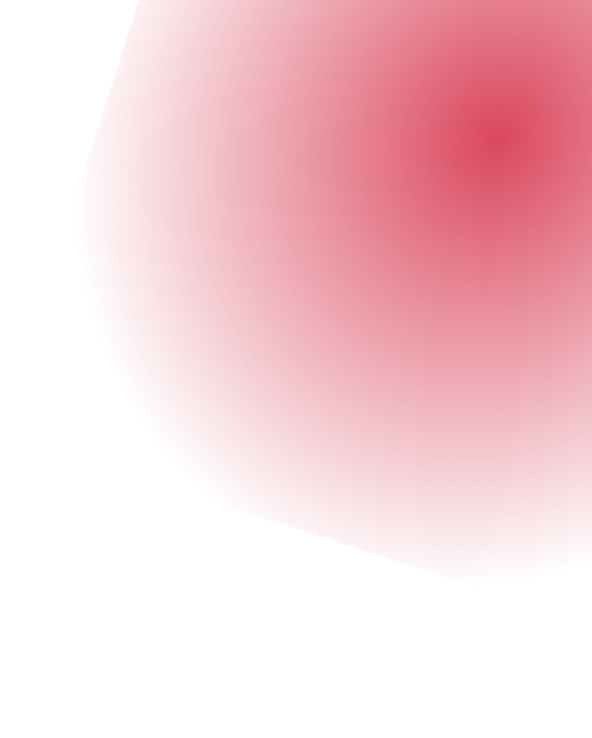
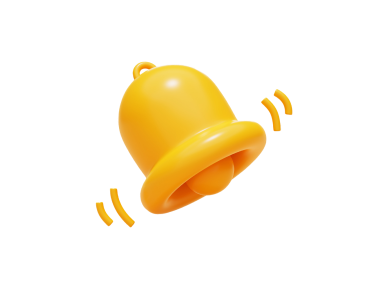
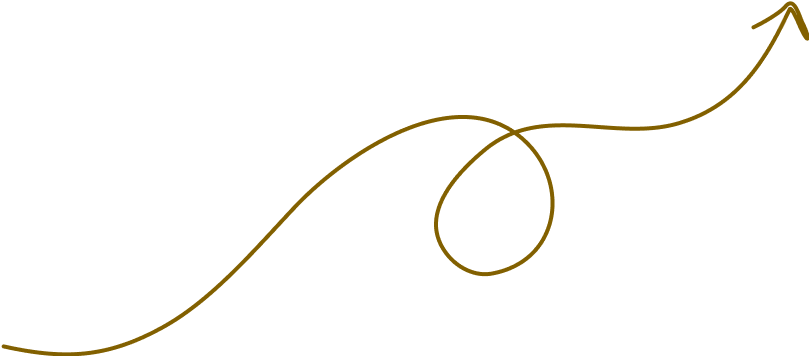